Smoother Image Scaling in NSTableView Cells
By default, NSImageCell's scaling strategy can produce some unpleasantly sharp edges. I looked at a bunch of different solutions to this using the existing API, but finally settled on a simple subclass.You can download it here, but here's the gist of it:
...
// make resizing look pretty
NSRect rect = NSMakeRect ( 0, 0, size.width, size.height );
NSImage * temp = [[NSImage alloc] initWithSize:rect.size];
[temp lockFocus];
[[NSGraphicsContext currentContext]
setImageInterpolation: NSImageInterpolationHigh];
[temp unlockFocus];
// resize image
NSPoint point = NSMakePoint( startX, startY );
[myImage setSize: size];
[myImage setScalesWhenResized: YES];
// draw image in cell
[myImage compositeToPoint:point
operation:NSCompositeSourceOver];
[temp release];
...
The results are pretty significant. Here's a before and after:
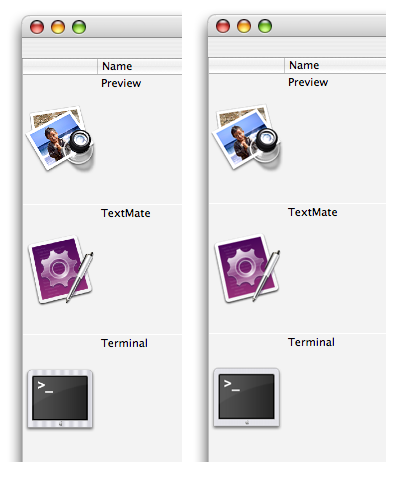
Here's a closeup:
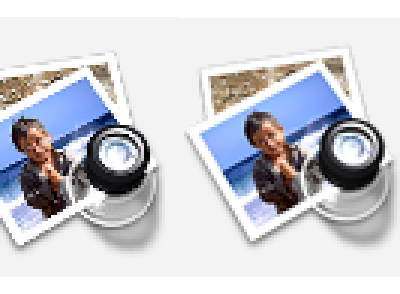
I recommend not selecting this as a custom subclass directly in Interface Builder, but instead do this in the controller:
- (void) awakeFromNib
{
NSTableColumn *thumbColumn;
thumbColumn = [myTable tableColumnWithIdentifier:@"thumbnail"];
THIImageCell * myImageCell = [[THIImageCell alloc] init];
[iconColumn setDataCell: myImageCell];
[myImageCell setImageAlignment:NSImageAlignCenter];
}
This should allow you to continue using NSImageCell's binding options through IB. Maybe it would work anyway, but I didn't try it.
I make no claims about this being the most efficient method available. If someone has suggestions for refinements, let me know.

Smoother Image Scaling in NSTableView Cells
Posted Nov 14, 2005 — 5 comments below
Posted Nov 14, 2005 — 5 comments below
Jean-Daniel Guyot — Nov 14, 05 548
Thanks for this helpful hint!
Damn Man — Nov 14, 05 549
copyright notice, this list of conditions and the following
disclaimer in the documentation and/or other materials provided
with the distribution.
I have no materials. I'll have to write my own version of the code. Bummer. Thanks for the tip, though.
DF — Nov 14, 05 550
Scott Stevenson — Nov 14, 05 551
It's just a standard BSD-derived license -- the same one I released DataCrux under. It seems exceptionally flexible to me, but I'll keep your comments in mind.
Buzz Andersen — Nov 14, 05 552